You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Copy file name to clipboardexpand all lines: 6-text-generation-apps/README.md
+44-35
Original file line number
Diff line number
Diff line change
@@ -20,15 +20,6 @@ At the end of this lesson, you'll be able to:
20
20
- Build a text generation app using openai.
21
21
- Configure your app to use more or less tokens and also change the temperature, for a varied output.
22
22
23
-
## References
24
-
25
-
TODO, openai
26
-
27
-
## Learning goals
28
-
29
-
- Understand what a text generation app is.
30
-
- Explain what semantic kernel is and how you can use it to build a text generation app.
31
-
32
23
## What is a text generation app?
33
24
34
25
Normally when you build an app it some kind of interface like the following:
@@ -76,47 +67,67 @@ Then there are libraries that operate on a higher level like:
76
67
-**Semantic Kernel**. Semantic Kernel is a library by Microsoft supporting the languages C#, Python, and Java.
77
68
78
69
## First app using openai
70
+
79
71
Let's see how we can build our first app, what libraries we need, how much is required and so on.
72
+
80
73
### Install openai
74
+
81
75
There are many libraries out there for interacting with OpenAI or Azure OpenAI. It's possible to use numerous programming languages as well like C#, Python, JavaScript, Java and more. We've chosen to use the `openai` Python library, so we'll use `pip` to install it.
76
+
82
77
```bash
83
78
pip install openai
84
79
```
85
80
86
-
### Create an account
81
+
### Create a resource
87
82
88
-
Go to https://beta.openai.com/ and create an account.
83
+
You need to carry out the following steps:
89
84
90
-
TODO, add instructions for Azure.
85
+
- Create an account on Azure <https://azure.microsoft.com/free/>.
86
+
- Gain access to Azure Open AI. Go to <https://learn.microsoft.com/en-us/azure/ai-services/openai/overview#how-do-i-get-access-to-azure-openai> and request access.
91
87
92
-
### Setup your API key
88
+
> [!NOTE]
89
+
> At the time of writing, you need to apply for access to Azure Open AI.
93
90
94
-
You can use one of two ways to setup your API key:
91
+
- Install Python <https://www.python.org/>
92
+
- Have created an Azure OpenAI Service resource. See this guide for how to [create a resource](https://learn.microsoft.com/en-us/azure/ai-services/openai/how-to/create-resource?pivots=web-portal).
95
93
96
-
- Set the environment variable `OPENAI_API_KEY` to your API key.
97
94
98
-
```bash
99
-
export OPENAI_API_KEY='sk-...'
100
-
```
101
-
- Set it in code:
95
+
### Locate API key and endpoint
102
96
103
-
```python
104
-
import openai
105
-
openai.api_key = "sk-..."
106
-
```
97
+
At this point, you need to tell your `openai` library what API key to use. To find your API key, go to "Keys and Endpoint" section of your Azure Open AI resource and copy the "Key 1" value.
107
98
108
-
Once you're setup with your API key, you can start using the library to generate text.
99
+
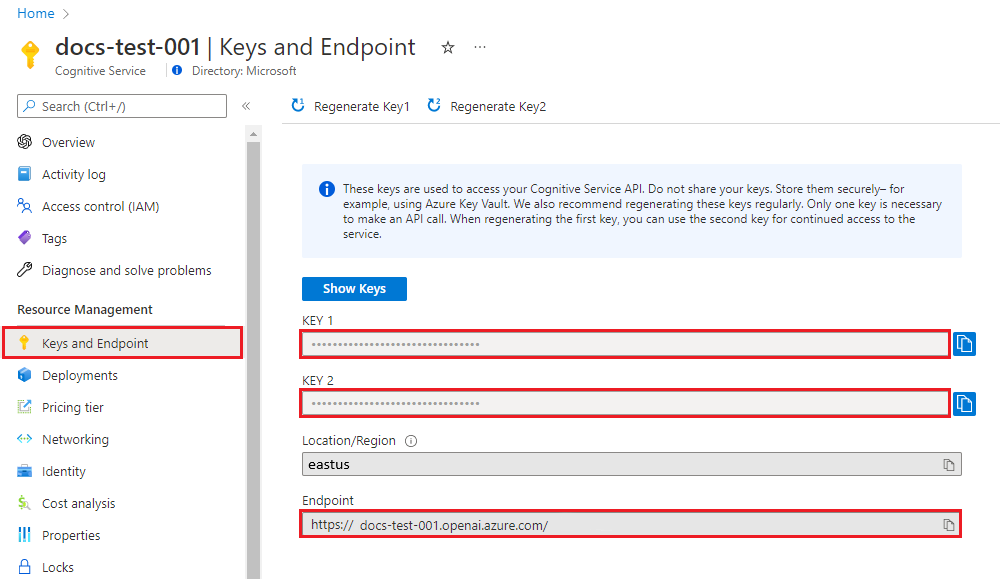
109
100
110
-
If you're using Azure Open AI, here's how setup configuration:
101
+
Now that you have this information copied, let's instruct the libraries to use it.
111
102
112
-
```python
113
-
import openai
103
+
> [!NOTE]
104
+
> It's worth separating your API key from your code. You can do so by using environment variables.
105
+
> - Set the environment variable `OPENAI_API_KEY` to your API key.
106
+
> `export OPENAI_API_KEY='sk-...'`
107
+
108
+
109
+
### Setup configuration Azure
114
110
111
+
If you're using Azure Open AI, here's how you setup configuration:
112
+
113
+
```python
115
114
openai.api_type ='azure'
116
115
openai.api_key = os.environ["OPENAI_API_KEY"]
116
+
openai.api_version ='2023-05-15'
117
+
openai.api_base = os.getenv("API_BASE")
117
118
```
118
119
119
-
### Generate text
120
+
Above we're setting the following:
121
+
122
+
-`api_type` to `azure`. This tells the library to use Azure Open AI and not OpenAI.
123
+
-`api_key`, this is your API key found in the Azure Portal.
124
+
-`api_version`, this is the version of the API you want to use. At the time of writing, the latest version is `2023-05-15`.
125
+
-`api_base`, this is the endpoint of the API. You can find it in the Azure Portal next to your API key.
126
+
127
+
> [!NOTE]
128
+
> `os.getenv` is a function that reads environment variables. You can use it to read environment variables like `OPENAI_API_KEY` and `API_BASE`. Set these environment variables in your terminal or by using a library like `dotenv`.
129
+
130
+
## Generate text
120
131
121
132
The way to generate text is to use the `Completion` class. Here's an example:
122
133
@@ -168,19 +179,17 @@ Now that we learned how to setup and configure openai, it's time to build your f
168
179
import openai
169
180
170
181
openai.api_key = "<replace this value with your open ai key or Azure Open AI key>"
171
-
# azure
172
182
173
-
# enable below if you use Azure Open AI
174
-
# openai.api_type = 'azure'
175
-
# openai.api_version = '2023-05-15'
176
-
# openai.api_base = "<endpoint found in Azure Portal where your API key is>"
177
-
183
+
openai.api_type = 'azure'
184
+
openai.api_version = '2023-05-15'
185
+
openai.api_base = "<endpoint found in Azure Portal where your API key is>"
186
+
deployment_name = "<deployment name>"
178
187
179
188
# add your completion code
180
189
prompt = "Complete the following: Once upon a time there was a"
0 commit comments