|
| 1 | +--- |
| 2 | +title: "Supabase: The Open Source Firebase Alternative" |
| 3 | +description: Supabase is an open-source Firebase alternative that provides a suite of tools for building scalable applications without the need to write a backend. It leverages PostgreSQL for real-time databases, offers authentication, and file storage capabilities. Supabase aims to democratize backend development by providing a modern, scalable, and open-source platform. |
| 4 | +author: Lohith Lalam |
| 5 | +lastmod: 2023-06-15 |
| 6 | +publishdate: 2023-06-15 |
| 7 | +tags: |
| 8 | + - supabase |
| 9 | + - database |
| 10 | + - introduction |
| 11 | + |
| 12 | +draft: false |
| 13 | +--- |
| 14 | + |
| 15 | +## Table of Contents |
| 16 | + |
| 17 | +- [Table of Contents](#table-of-contents) |
| 18 | +- [Introduction](#introduction) |
| 19 | +- [Setup](#setup) |
| 20 | + - [Prerequisites](#prerequisites) |
| 21 | + - [Steps](#steps) |
| 22 | +- [Tutorial with an Example Project](#tutorial-with-an-example-project) |
| 23 | + - [Example Project: Todo List Application](#example-project-todo-list-application) |
| 24 | + - [Steps](#steps-1) |
| 25 | +- [Features and Links](#features-and-links) |
| 26 | +- [List of Projects to Do Using Supabase](#list-of-projects-to-do-using-supabase) |
| 27 | +- [List of Projects That Use Supabase](#list-of-projects-that-use-supabase) |
| 28 | +- [Making the Best of Supabase](#making-the-best-of-supabase) |
| 29 | +- [Conclusion](#conclusion) |
| 30 | + |
| 31 | +## Introduction |
| 32 | + |
| 33 | +Supabase is an open-source Firebase alternative that provides a suite of tools for building scalable applications without the need to write a backend. It leverages PostgreSQL for real-time databases, offers authentication, and file storage capabilities. Supabase aims to democratize backend development by providing a modern, scalable, and open-source platform. |
| 34 | + |
| 35 | +## Setup |
| 36 | + |
| 37 | +### Prerequisites |
| 38 | + |
| 39 | +- Node.js installed on your computer. |
| 40 | +- A web browser. |
| 41 | +- A text editor or IDE like VSCode. |
| 42 | + |
| 43 | +### Steps |
| 44 | + |
| 45 | +1. **Sign Up for Supabase** |
| 46 | + - Visit [Supabase](https://supabase.io) and sign up for a free account. |
| 47 | + - Once logged in, create a new project. |
| 48 | + |
| 49 | +2. **Create a New Project** |
| 50 | + - In the Supabase dashboard, click on "New Project". |
| 51 | + - Provide a name for your project, choose a database password, and select a region. |
| 52 | + |
| 53 | +<div align="center"> |
| 54 | + |
| 55 | +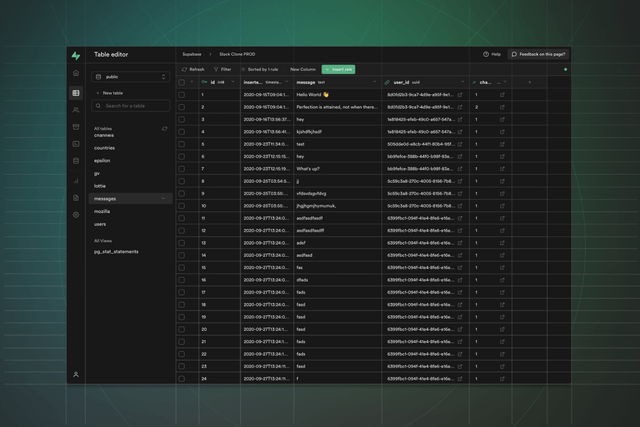 |
| 56 | +</div> |
| 57 | + |
| 58 | +3. **Configure Your Database** |
| 59 | + - After creating the project, you’ll be directed to the project’s overview page. |
| 60 | + - Note down the API URL and the API key, as you’ll need these for your frontend application. |
| 61 | + |
| 62 | +## Tutorial with an Example Project |
| 63 | + |
| 64 | +### Example Project: Todo List Application |
| 65 | + |
| 66 | +In this tutorial, we’ll build a simple Todo List application using Supabase and React. |
| 67 | + |
| 68 | +### Steps |
| 69 | + |
| 70 | +1. **Initialize a React Application** |
| 71 | + - Open your terminal and run the following command to create a new React application: |
| 72 | + |
| 73 | + ```bash |
| 74 | + npx create-react-app supabase-todo |
| 75 | + cd supabase-todo |
| 76 | + ``` |
| 77 | + |
| 78 | +2. **Install Supabase Client** |
| 79 | + - Inside your React project directory, install the Supabase client: |
| 80 | + |
| 81 | + ```bash |
| 82 | + npm install @supabase/supabase-js |
| 83 | + ``` |
| 84 | + |
| 85 | +3. **Set Up Supabase Client** |
| 86 | + - Create a new file named `supabaseClient.js` in the `src` directory and add the following code: |
| 87 | + |
| 88 | + ```javascript |
| 89 | + import { createClient } from '@supabase/supabase-js'; |
| 90 | +
|
| 91 | + const supabaseUrl = 'https://your-supabase-url.supabase.co'; |
| 92 | + const supabaseKey = 'your-anon-key'; |
| 93 | +
|
| 94 | + export const supabase = createClient(supabaseUrl, supabaseKey); |
| 95 | + ``` |
| 96 | +
|
| 97 | +4. **Create a Database Table** |
| 98 | + - In the Supabase dashboard, go to the SQL editor and run the following SQL to create a `todos` table: |
| 99 | +
|
| 100 | + ```sql |
| 101 | + create table todos ( |
| 102 | + id bigint generated by default as identity primary key, |
| 103 | + task text not null, |
| 104 | + is_complete boolean default false |
| 105 | + ); |
| 106 | + ``` |
| 107 | +
|
| 108 | +5. **Fetch Todos from Supabase** |
| 109 | + - In your `App.js` file, import Supabase and fetch the todos: |
| 110 | +
|
| 111 | + ```javascript |
| 112 | + import React, { useState, useEffect } from 'react'; |
| 113 | + import { supabase } from './supabaseClient'; |
| 114 | +
|
| 115 | + function App() { |
| 116 | + const [todos, setTodos] = useState([]); |
| 117 | +
|
| 118 | + useEffect(() => { |
| 119 | + fetchTodos(); |
| 120 | + }, []); |
| 121 | +
|
| 122 | + const fetchTodos = async () => { |
| 123 | + let { data: todos, error } = await supabase |
| 124 | + .from('todos') |
| 125 | + .select('*'); |
| 126 | + if (error) console.log('Error:', error); |
| 127 | + else setTodos(todos); |
| 128 | + }; |
| 129 | +
|
| 130 | + return ( |
| 131 | + <div className="App"> |
| 132 | + <h1>Todo List</h1> |
| 133 | + <ul> |
| 134 | + {todos.map(todo => ( |
| 135 | + <li key={todo.id}>{todo.task}</li> |
| 136 | + ))} |
| 137 | + </ul> |
| 138 | + </div> |
| 139 | + ); |
| 140 | + } |
| 141 | +
|
| 142 | + export default App; |
| 143 | + ``` |
| 144 | +
|
| 145 | +6. **Add a New Todo** |
| 146 | + - Add a form to your `App.js` to allow adding new todos: |
| 147 | +
|
| 148 | + ```javascript |
| 149 | + function App() { |
| 150 | + const [todos, setTodos] = useState([]); |
| 151 | + const [task, setTask] = useState(''); |
| 152 | +
|
| 153 | + useEffect(() => { |
| 154 | + fetchTodos(); |
| 155 | + }, []); |
| 156 | +
|
| 157 | + const fetchTodos = async () => { |
| 158 | + let { data: todos, error } = await supabase |
| 159 | + .from('todos') |
| 160 | + .select('*'); |
| 161 | + if (error) console.log('Error:', error); |
| 162 | + else setTodos(todos); |
| 163 | + }; |
| 164 | +
|
| 165 | + const addTodo = async (task) => { |
| 166 | + let { data: todo, error } = await supabase |
| 167 | + .from('todos') |
| 168 | + .insert([{ task }]); |
| 169 | + if (error) console.log('Error:', error); |
| 170 | + else fetchTodos(); |
| 171 | + }; |
| 172 | +
|
| 173 | + return ( |
| 174 | + <div className="App"> |
| 175 | + <h1>Todo List</h1> |
| 176 | + <form onSubmit={(e) => { |
| 177 | + e.preventDefault(); |
| 178 | + addTodo(task); |
| 179 | + setTask(''); |
| 180 | + }}> |
| 181 | + <input |
| 182 | + type="text" |
| 183 | + value={task} |
| 184 | + onChange={(e) => setTask(e.target.value)} |
| 185 | + placeholder="Add a new task" |
| 186 | + /> |
| 187 | + <button type="submit">Add</button> |
| 188 | + </form> |
| 189 | + <ul> |
| 190 | + {todos.map(todo => ( |
| 191 | + <li key={todo.id}>{todo.task}</li> |
| 192 | + ))} |
| 193 | + </ul> |
| 194 | + </div> |
| 195 | + ); |
| 196 | + } |
| 197 | +
|
| 198 | + export default App; |
| 199 | + ``` |
| 200 | +
|
| 201 | +<div align="center"> |
| 202 | +
|
| 203 | +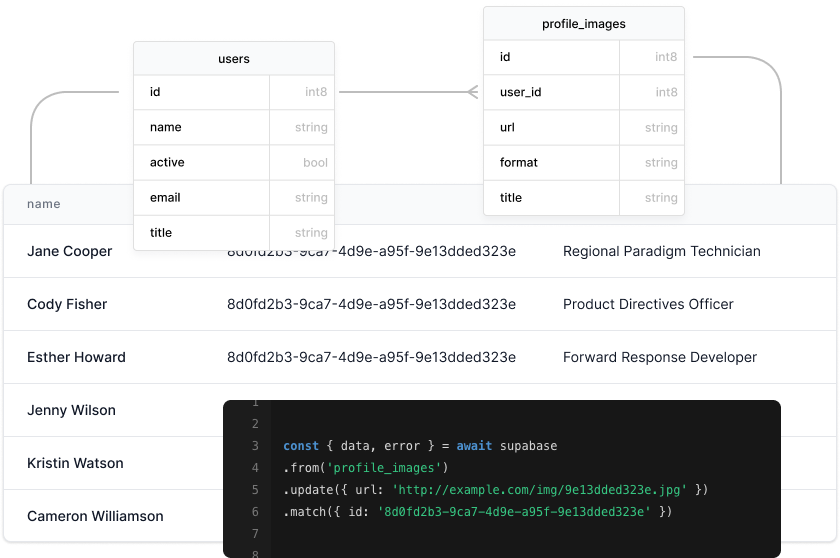 |
| 204 | +</div> |
| 205 | +
|
| 206 | +## Features and Links |
| 207 | +
|
| 208 | +Supabase offers various features including: |
| 209 | +
|
| 210 | +- **Real-Time Database**: Based on PostgreSQL with real-time capabilities. |
| 211 | +- **Authentication**: Support for multiple authentication methods. |
| 212 | +- **File Storage**: Secure storage for large files. |
| 213 | +- **API Generation**: Automatic RESTful API generation. |
| 214 | +
|
| 215 | +For more detailed information and guides, visit the [Supabase documentation](https://supabase.io/docs). |
| 216 | +
|
| 217 | +## List of Projects to Do Using Supabase |
| 218 | +
|
| 219 | +- **Chat Application**: Real-time messaging with user authentication. |
| 220 | +- **E-commerce Platform**: Product listings, user accounts, and real-time inventory management. |
| 221 | +- **Project Management Tool**: Collaborative project management with real-time updates. |
| 222 | +- **Social Media App**: User profiles, posts, and comments with real-time interactions. |
| 223 | +
|
| 224 | +## List of Projects That Use Supabase |
| 225 | +
|
| 226 | +- **[GitHub Finder](https://github.com/yourgithubusername/github-finder)** |
| 227 | +- **[Real-Time Chat](https://github.com/yourgithubusername/realtime-chat)** |
| 228 | +- **[Task Manager](https://github.com/yourgithubusername/task-manager)** |
| 229 | +- **[E-commerce Store](https://github.com/yourgithubusername/ecommerce-store)** |
| 230 | +
|
| 231 | +## Making the Best of Supabase |
| 232 | +
|
| 233 | +- **Utilize Real-Time Features**: Leverage real-time capabilities for interactive applications. |
| 234 | +- **Secure Authentication**: Implement robust user authentication and authorization workflows. |
| 235 | +- **Explore Supabase Community**: Engage with the community for support, ideas, and collaboration. |
| 236 | +- **Stay Updated**: Keep an eye on new features and updates from the Supabase team. |
| 237 | +
|
| 238 | +## Conclusion |
| 239 | +
|
| 240 | +Supabase is a powerful and open-source alternative to Firebase, offering real-time databases, authentication, and file storage with the robustness of PostgreSQL. Its ease of integration, scalability, and cost-effective pricing make it an excellent choice for modern application development. |
| 241 | +
|
| 242 | +By following this guide, you can quickly get started with Supabase and build a variety of applications efficiently. Explore the Supabase ecosystem and leverage its capabilities to create innovative and responsive applications. |
0 commit comments